First we give the schema user scott permission to create a queue.
grant aq_user_role to scott;
grant execute on DBMS_AQADM to scott;
We can create the queue table
begin
sys.dbms_aqadm.create_queue_table(
queue_table => 'JMS_IN_TABLE',
queue_payload_type => 'SYS.AQ$_JMS_MESSAGE',
sort_list => 'PRIORITY, ENQ_TIME',
compatible => '10.0.0',
primary_instance => 0,
secondary_instance => 0,
storage_clause => 'tablespace users pctfree 10 initrans 1 maxtrans 255 storage ( initial 64K minextents 1 maxextents unlimited )');
end;
/
What important is that we use 'SYS.AQ$_JMS_MESSAGE' as payload type and the dequeue order is first priority and then the enqueue time.
Now we can create the queue
begin
sys.dbms_aqadm.create_queue(
queue_name => 'JMS_IN',
queue_table => 'JMS_IN_TABLE',
queue_type => sys.dbms_aqadm.normal_queue);
end;
/
If you want to dequeue and enqueue this queue we have to enable this first
begin
dbms_aqadm.start_queue( queue_name =>'JMS_IN' ,enqueue => true ,dequeue => true );
end;
/
With this plsql code we can add a message to the queue
declare
l_message clob := '';
v_enqueue_options dbms_aq.enqueue_options_t;
v_msg_props dbms_aq.message_properties_t;
v_msg_id RAW(16);
v_message SYS.AQ$_JMS_MESSAGE := SYS.AQ$_JMS_MESSAGE.construct(DBMS_AQ.JMS_TEXT_MESSAGE);
begin
l_message := '<data-message><runid>210</runid></data-message>';
v_message.set_text(xmltype(l_message).getstringval());
dbms_aq.enqueue(queue_name => 'JMS_IN'
,enqueue_options => v_enqueue_options
,message_properties => v_msg_props
,payload => v_message
,msgid => v_msg_id);
commit;
end;
/
Now we are ready to use this in the ESB. We can add a new JMS adapter in the composite view.
Choose database as oems jms provider
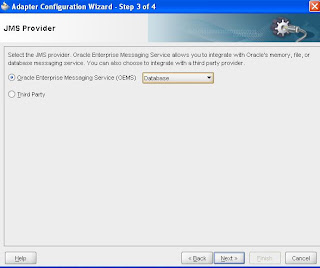
Create a new database connection to scott.
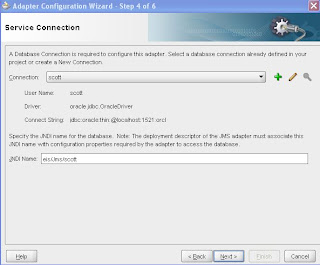
If you want to enqueue a message then choose produce and if you want to dequeue choose consumer
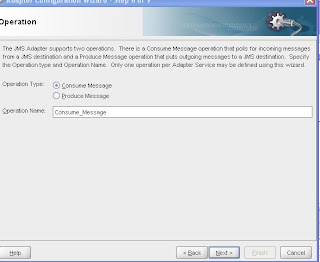
Give the resource provider a name ( we need this name later )and select the destination.
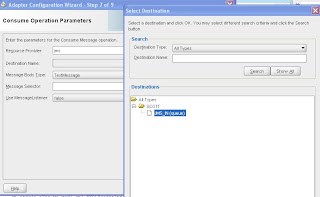
Now we add the resource provider in the application.xml in the config folder, use the same name as above
<resource-provider class="oracle.jms.OjmsContext" name="jms">
<description>oc4j-jms loop back resource provider</description>
<property name="url" value="jdbc:oracle:thin:scott/tiger@localhost:1521:orcl"/>
</resource-provider>
The last step is to configure the jms adapter. Go to the deployment folder then to the default and JmsAdapter folder. Here we edit the oc4j-ra.xml where we add a new connection
Location is the database connection name and change the user and password properties. We have to change the value of connectionFactoryLocation property. Between /resource and /QueueConnectionFactoryLocation we have to add the resource provider name.
<connection-factory connector-name="Jms Adapter" location="eis/Jms/scott">
<config-property name="connectionFactoryLocation" value="java:comp/resource/jms/QueueConnectionFactories/myQCF" encoded-credential="false"/>
<config-property name="factoryProperties" value="" encoded-credential="false"/>
<config-property name="acknowledgeMode" value="AUTO_ACKNOWLEDGE" encoded-credential="false"/>
<config-property name="isTopic" value="false" encoded-credential="false"/>
<config-property name="isTransacted" value="true" encoded-credential="false"/>
<config-property name="username" value="scott" encoded-credential="false"/>
<config-property name="password" value="tiger" encoded-credential="false"/>
</connection-factory>
Now we can use the jms queue in the esb.
Thank you, helped me very much with creation of new AQ messages.
ReplyDelete