In a
earlier post I already made Adobe Flex / BlazeDS / EJB2.1 example and this example was deployed on an OC4J J2EE container. In this post I let the same example run in Oracle Weblogic 10.3.2 ( FMW11g) with the BlazeDS jars as a shared library ( this also works with Adobe Lifecycle). Also in the earlier post I needed to use EJB2.1 but with EJB factory of Peter Martin I can use Eclipselink.
To start, we need to download
BlazeDS and
EJB and Flex Integration jar, Extract these archives so we can copy the jar files to a new location.
We need to make a shared library for weblogic. This is better then just add all the jars to WEB-INF/lib folder of every web application. You can patch the blazeds jars without re-deploying your webapps and you now know exactly which version of blazeds you are using.
Make a folder called blazeds with as subfolders APP-INF and META-INF and add a empty zip in it and call it empty.jar
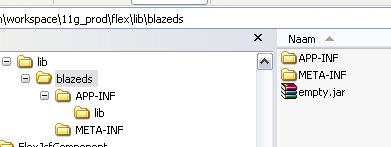
Copy the blazeds and ejb factory jars in the APP-INF/lib folder
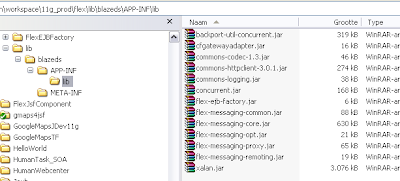
In the META-INF folder we need to add two files application.xml and MANIFEST.MF where we will add the library information for weblogic.
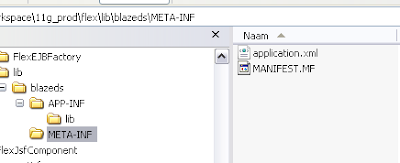
The application.xml
<?xml version = '1.0' encoding="UTF-8" ?>
<application xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
version="1.4">
<description>BlazeDS Library</description>
<display-name>blazeds</display-name>
<module>
<java>empty.jar</java>
</module>
</application>
The MANIFEST.MF has this content
Manifest-Version: 1.0
Ant-Version: Apache Ant 1.7.0RC1
Created-By: 14.0-b16 (Sun Microsystems Inc.)
Extension-Name: blazeds
Specification-Version: 3.2
Implementation-Title: BlazeDS - BlazeDS Application
Implementation-Version: 3.2.0.3978
Implementation-Vendor: Adobe Systems Inc.
We are ready to add this library to the Weblogic Server. The library folder is on the same file system as the weblogic server so I don't need to make an EAR file, I can just use the exploded folder. Open the Weblogic Console and go to Deployments. Here we can install a new Deployment.
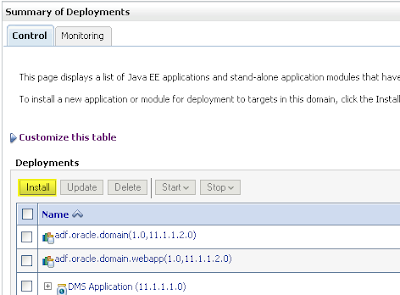
Go to blazeds library folder
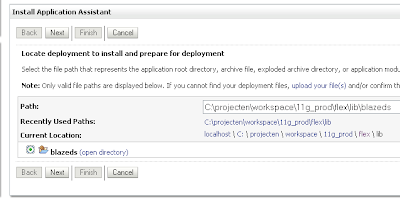
Install it as a libray
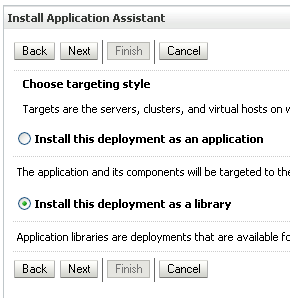
Weblogic will detect the manifest and application.xml and press Finish
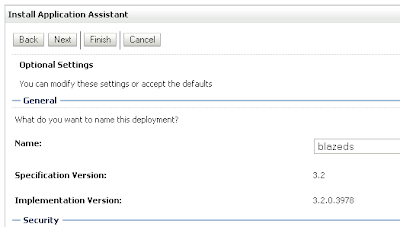
In the deployment page we can see the blazeds library.
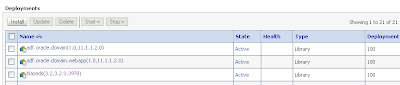
In the ViewController project we need to make a reference to this shared libray, to do this add a weblogic deployment descriptor. We can use weblogic-application.xml located in the META-INF folder of the EAR or use weblogic.xml in the WEB-INF of the Viewcontroller project. Here is an example of my weblogic-application.xml
<?xml version = '1.0' encoding = 'windows-1252'?>
<weblogic-application xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.bea.com/ns/weblogic/weblogic-application http://www.bea.com/ns/weblogic/weblogic-application/1.0/weblogic-application.xsd"
xmlns="http://www.bea.com/ns/weblogic/weblogic-application">
<library-ref>
<library-name>blazeds</library-name>
</library-ref>
</weblogic-application>
The last steps is to configure the web.xml for blazeds and the blazeds configuration files for the EJB call.
first the web.xml of the viewcontroller project.
<?xml version = '1.0' encoding = 'windows-1252'?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
version="2.5" xmlns="http://java.sun.com/xml/ns/javaee">
<description>Empty web.xml file for Web Application</description>
<servlet>
<servlet-name>MessageBrokerServlet</servlet-name>
<servlet-class>flex.messaging.MessageBrokerServlet</servlet-class>
<init-param>
<param-name>services.configuration.file</param-name>
<param-value>/WEB-INF/flex/services-config.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>MessageBrokerServlet</servlet-name>
<url-pattern>/messagebroker/*</url-pattern>
</servlet-mapping>
<listener>
<listener-class>flex.messaging.HttpFlexSession</listener-class>
</listener>
<mime-mapping>
<extension>html</extension>
<mime-type>text/html</mime-type>
</mime-mapping>
<mime-mapping>
<extension>txt</extension>
<mime-type>text/plain</mime-type>
</mime-mapping>
</web-app>
I use the remoting-config.xml and services-config.xml files both located in WEB-INF/flex folder
Here is my remoting-config.xml file, where source element is the JNDI name of my EJB Session Bean, after deployed of your model you can look it up in the Weblogic console ( servers, select the server and in the top there is a link to the jndi page )
<?xml version="1.0" encoding="UTF-8"?>
<service id="remoting-service" class="flex.messaging.services.RemotingService">
<adapters>
<adapter-definition id="java-object"
class="flex.messaging.services.remoting.adapters.JavaAdapter"
default="true"/>
</adapters>
<default-channels>
<channel ref="my-amf"/>
</default-channels>
<destination id="EmployeeEJB">
<properties>
<factory>ejb3</factory>
<source>flex_ejb2-Model-HRSessionEJB#nl.whitehorses.model.services.HRSessionEJB</source>
</properties>
</destination>
</service>
my services-config.xml
<?xml version="1.0" encoding="UTF-8"?>
<services-config>
<services>
<service-include file-path="remoting-config.xml"/>
<default-channels>
<channel ref="my-amf"/>
</default-channels>
</services>
<factories>
<factory id="ejb3" class="com.adobe.ac.ejb.EJB3Factory"/>
</factories>
<channels>
<channel-definition id="my-amf"
class="mx.messaging.channels.AMFChannel">
<endpoint url="http://{server.name}:{server.port}/{context.root}/messagebroker/amf"
class="flex.messaging.endpoints.AMFEndpoint"/>
<properties>
<polling-enabled>false</polling-enabled>
</properties>
</channel-definition>
</channels>
<logging>
<target class="flex.messaging.log.ConsoleTarget" level="Error">
<properties>
<prefix>[Flex]</prefix>
<includeDate>false</includeDate>
<includeTime>false</includeTime>
<includeLevel>false</includeLevel>
<includeCategory>false</includeCategory>
</properties>
</target>
</logging>
</services-config>
Deploy everything to the Weblogic server.
and at last the Adobe mxml where I call the getEmployeesFindAll method.
<?xml version="1.0" encoding="utf-8"?>
<mx:Application xmlns:mx="http://www.adobe.com/2006/mxml" width="1155" height="206.21213">
<mx:applicationComplete>srv.getEmployeesFindAll()</mx:applicationComplete>
<mx:RemoteObject id="srv" showBusyCursor="true" destination="EmployeeEJB"/>
<mx:DataGrid width="1104.6212" dataProvider="{srv.getEmployeesFindAll.lastResult}" height="140.98485"/>
</mx:Application>