The user have to authenticate in the Customer application and this user is also known in the Human Task ( I add the same authenticator in the ADF & Soa Suite server) So why don't we use the same user for the EJB call to the Soa Suite.
To make this works you need to make a domain trust between the two Weblogic domains.
To do this you need to go to the weblogic console and change some domain properties.
First change, enable the "Cross Domain Security Enabled" option.
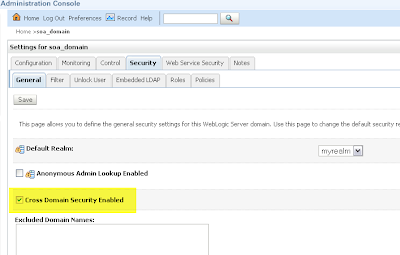
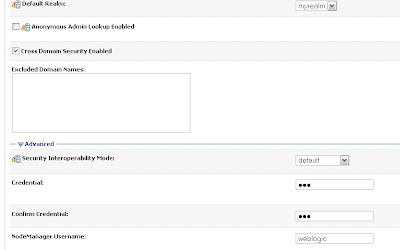
Do this on both domains.
The next step is to create Workflow Client in your application. It will use your Application authentication for this remote EJB, so you don't to have a Soa Suite account in your application code.
String wlsserver = "HumanWorkFlow";
String soaserver = System.getProperty("humantask.url");
String wsurl = "http://"+soaserver;
String t3url = "t3://"+soaserver;
String contextFactory = "weblogic.jndi.WLInitialContextFactory";
String identityDomain = "jazn.com";
IWorkflowContext context = null;
IWorkflowServiceClient workflowServiceClient;
BPMIdentityService bpmClient;
WorkflowServicesClientConfigurationType wscct = new WorkflowServicesClientConfigurationType();
List<ServerType> servers = wscct.getServer();
ServerType server = new ServerType();
server.setDefault(true);
server.setName(wlsserver);
servers.add(server);
RemoteClientType rct = new RemoteClientType();
rct.setServerURL(t3url);
rct.setInitialContextFactory(contextFactory);
rct.setParticipateInClientTransaction(false);
server.setRemoteClient(rct);
workflowServiceClient = WorkflowServiceClientFactory.getWorkflowServiceClient(
WorkflowServiceClientFactory.REMOTE_CLIENT,
wscct,
logger2);
Map<IWorkflowServiceClientConstants.CONNECTION_PROPERTY,java.lang.String> properties =
new HashMap<IWorkflowServiceClientConstants.CONNECTION_PROPERTY,java.lang.String>();
properties.put(IWorkflowServiceClientConstants.CONNECTION_PROPERTY.SOAP_END_POINT_ROOT
, wsurl);
bpmClient = WorkflowServiceClientFactory.getSOAPIdentityServiceClient(identityDomain
, properties
, logger2);
The last step is to acquire and release the Human Tasks on behalf of the application user. Because you don't know the application user password you need to have an account which can do that for the application user. This code can do that for the application user. Make sure that this account don't have too much authorization rights in Weblogic and your application.
String identityUsername = System.getProperty("humantask.user" );
String identityPassword = System.getProperty("humantask.password");
IWorkflowContext contextBehalf = null;
ITaskQueryService taskQueryService = getTaskQueryService();
if ( context == null ) {
System.out.println("HumanWorkflow "+identityUsername+ " context created");
context = taskQueryService.authenticate( identityUsername
, identityPassword.toCharArray()
, identityDomain);
}
contextBehalf = taskQueryService.authenticateOnBehalfOf(context, onBehalfOfUser);