This is how SAML Sender Vouches works and what we need to do in weblogic / java.
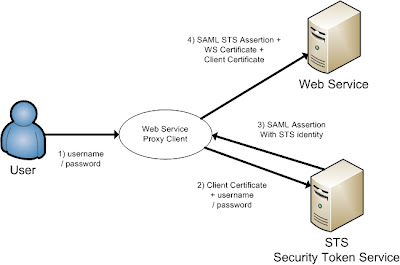
The long version, the user provides its credentials to the ws proxy client and the ws proxy client calls the STS server and provides the username / password of the user and the client key.
The STS validates the user and the ws proxy client certificate and the STS returns the STS identity assertion to the ws proxy client. The ws proxy client uses this STS assertion together with the ws client and ws server certificate to call the web service.
First we need to have 3 certificates, the first is alice, this will be used in the ws proxy client and the second certificate is bob, this will be used in the Weblogic web service server and the last we use wssipsts certificate for the Weblogic STS server. Add these keys into a java keystore.
Setting up the Secure Token Service (STS)
Create a new Weblogic 10.3.1 domain and start the admin server. First we need to enable SSL in the general tab of the server and then add our keystores in the keystore tab.
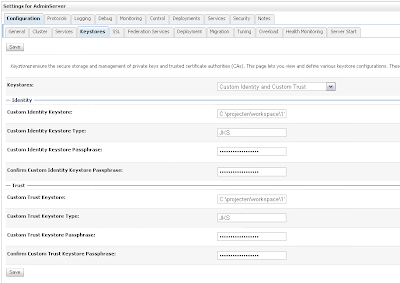
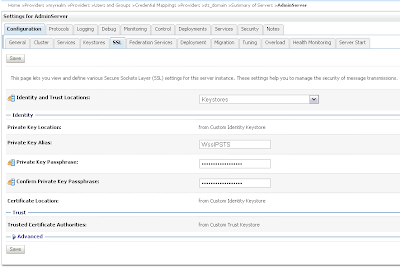
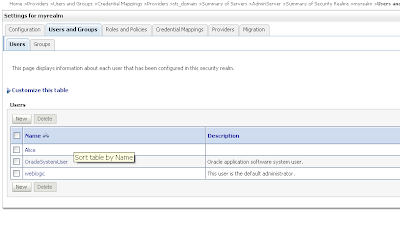
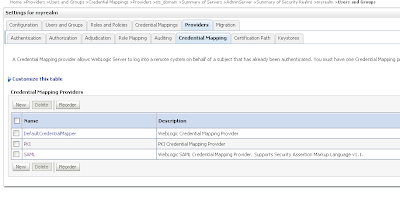
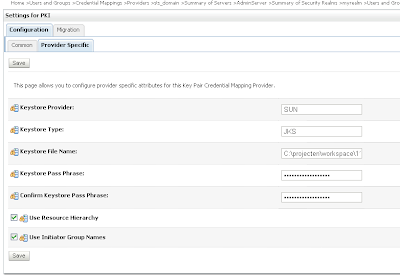
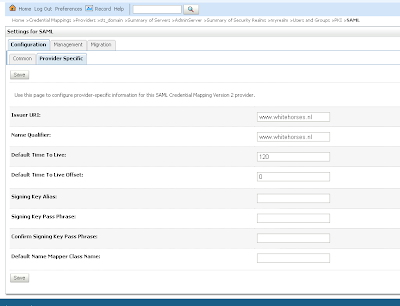
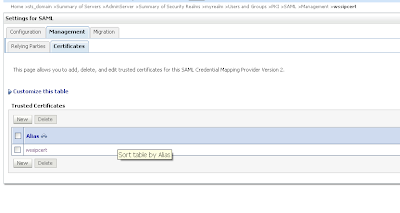
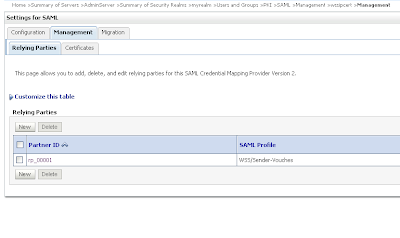
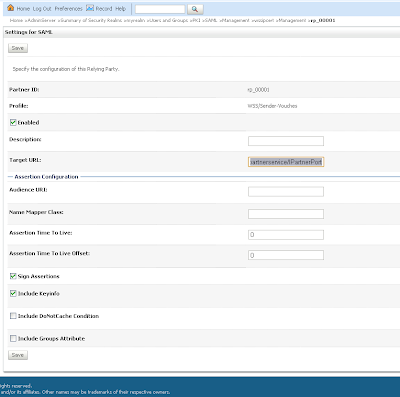
That is all for the STS server and now we can deploy the STS web service.
package nl.whitehorses.sts;
import weblogic.jws.Policy;
import weblogic.wsee.security.saml.SAMLTrustTokenProvider;
import weblogic.wsee.security.wst.framework.TrustTokenProviderRegistry;
import javax.jws.WebMethod;
import javax.jws.WebService;
@WebService
@Policy(uri="policy:Wssp1.2-2007-Wssc1.3-Bootstrap-Https-UNT.xml")
public class StsUnt {
static {
init();
}
@WebMethod
@Policy(uri="policy:Wssp1.2-2007-SignBody.xml")
public String dummyMethod(String s) {
return s;
}
static void init() {
TrustTokenProviderRegistry reg = TrustTokenProviderRegistry.getInstance();
SAMLTrustTokenProvider provider = new MySAMLTrustTokenProvider();
reg.registerProvider("http://docs.oasis-open.org/wss/2004/01/oasis-2004-01-saml-token-profile-1.0#SAMLAssertionID", provider);
reg.registerProvider("http://docs.oasis-open.org/wss/oasis-wss-saml-token-profile-1.0#SAMLAssertionID", provider);
reg.registerProvider("http://docs.oasis-open.org/wss/oasis-wss-saml-token-profile-1.1#SAMLV2.0", provider);
reg.registerProvider("http://docs.oasis-open.org/wss/oasis-wss-saml-token-profile-1.1#SAMLV1.0", provider);
reg.registerProvider("http://docs.oasis-open.org/wss/oasis-wss-saml-token-profile-1.1#SAMLV1.1", provider);
}
static class MySAMLTrustTokenProvider extends SAMLTrustTokenProvider {
}
}
Configure the Weblogic server for the Web Services
Create a new Weblogic domain and use the same keystore, we don't need to setup SSL on this server.
Go the myrealm security and go to providers tab where we add a new PKI Credential Mapping in the credentials tab. ( Use the same setting as the STS server )
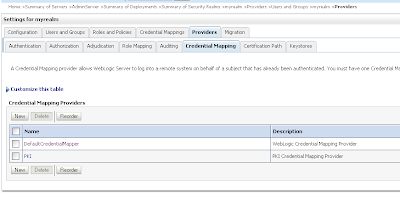
Create a SAML Authentication and SAML Identity Assertion provider.
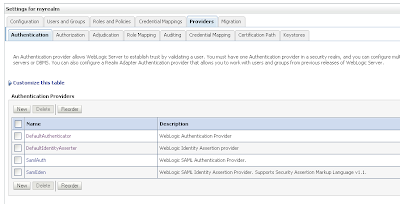
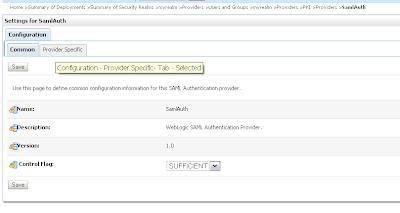
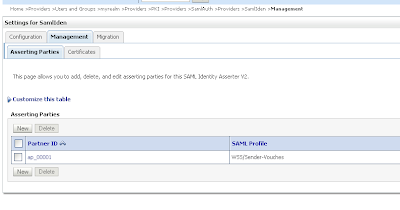
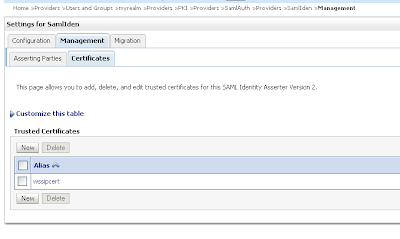
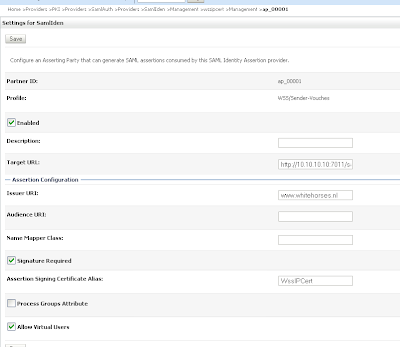
Change the defaultIdentityAsserter and add wsse:PasswordDigest and X.509 as active types.
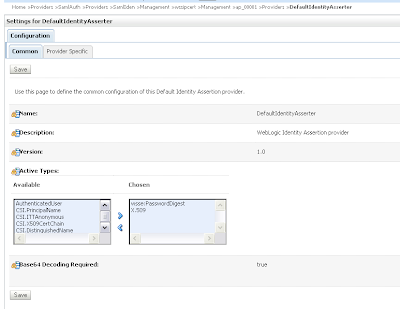
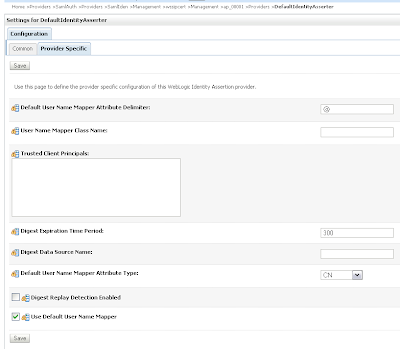
In the DefaultAuthenticator we need to set control flag to SUFFICIENT
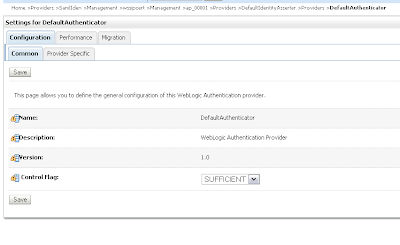
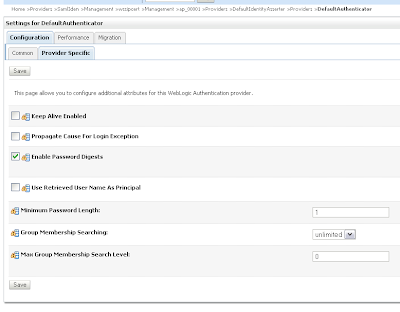
package nl.whitehorses.sts.ws;
import weblogic.jws.Policies;
import weblogic.jws.Policy;
import javax.jws.WebService;
@Policies(
{
@Policy(uri = "policy:Wssp1.2-2007-Saml1.1-SenderVouches-Wss1.0.xml"),
@Policy(uri = "policy:Wssp1.2-2007-SignBody.xml"),
@Policy(uri = "policy:Wssp1.2-2007-EncryptBody.xml")
}
)
@WebService
public class EchoService {
public String echo( String hello){
return hello;
}
}
Generating the Web Service Proxy Client
The last step we need to generate a web service proxy client and add the username and the client credentials mappings.
package nl.whitehorses.sts.ws.client;
import java.io.InputStream;
import java.io.ByteArrayInputStream;
import java.security.cert.X509Certificate;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.net.URL;
import javax.xml.namespace.QName;
import javax.xml.ws.BindingProvider;
import javax.xml.ws.WebServiceRef;
import weblogic.security.SSL.TrustManager;
import weblogic.wsee.message.WlMessageContext;
import weblogic.wsee.security.bst.ClientBSTCredentialProvider;
import weblogic.wsee.security.saml.SAMLTrustCredentialProvider;
import weblogic.wsee.security.unt.ClientUNTCredentialProvider;
import weblogic.xml.crypto.wss.WSSecurityContext;
import weblogic.xml.crypto.wss.provider.CredentialProvider;
public class EchoServicePortClient
{
@WebServiceRef
private static EchoServiceService echoServiceService;
private static String stsUntPolicy =
"<?xml version=\"1.0\"?>\n" +
"<wsp:Policy\n" +
" xmlns:wsp=\"http://schemas.xmlsoap.org/ws/2004/09/policy\"\n" +
" xmlns:sp=\"http://docs.oasis-open.org/ws-sx/ws-securitypolicy/200702\"\n" +
" >\n" +
" <sp:TransportBinding>\n" +
" <wsp:Policy>\n" +
" <sp:TransportToken>\n" +
" <wsp:Policy>\n" +
" <sp:HttpsToken/>\n" +
" </wsp:Policy>\n" +
" </sp:TransportToken>\n" +
" <sp:AlgorithmSuite>\n" +
" <wsp:Policy>\n" +
" <sp:Basic256/>\n" +
" </wsp:Policy>\n" +
" </sp:AlgorithmSuite>\n" +
" <sp:Layout>\n" +
" <wsp:Policy>\n" +
" <sp:Lax/>\n" +
" </wsp:Policy>\n" +
" </sp:Layout>\n" +
" <sp:IncludeTimestamp/>\n" +
" </wsp:Policy>\n" +
" </sp:TransportBinding>\n" +
" <sp:SupportingTokens>\n" +
" <wsp:Policy>\n" +
" <sp:UsernameToken\n" +
" sp:IncludeToken=\"http://docs.oasis-open.org/ws-sx/ws-securitypolicy/200702/IncludeToken/AlwaysToRecipient\">\n" +
" <wsp:Policy>\n" +
" <sp:WssUsernameToken10/>\n" +
" </wsp:Policy>\n" +
" </sp:UsernameToken>\n" +
" </wsp:Policy>\n" +
" </sp:SupportingTokens>\n" +
"</wsp:Policy>";
public static void main(String[] args) {
System.setProperty("com.sun.xml.ws.transport.http.client.HttpTransportPipe.dump", "true");
try {
String wsURL = "http://10.10.10.10:7011/saml-ws-context-root/EchoServicePort?WSDL";
echoServiceService = new EchoServiceService( new URL(wsURL)
, new QName("http://ws.sts.whitehorses.nl/", "EchoServiceService"));
EchoService echoService = echoServiceService.getEchoServicePort();
System.setProperty("javax.net.ssl.trustStore", "C:/projecten/workspace/11g_prod/saml1.1_ws/wsttest1/certs/cacerts");
Map<String, Object> requestContext = ((BindingProvider)echoService).getRequestContext();
List<CredentialProvider> credList = new ArrayList<CredentialProvider>();
// Add the necessary credential providers to the list
InputStream policy = new ByteArrayInputStream(stsUntPolicy.getBytes("UTF-8"));
requestContext.put(WlMessageContext.WST_BOOT_STRAP_POLICY, policy );
String stsURL = "https://localhost:7022/sts/StsUntPort";
requestContext.put(WlMessageContext.STS_ENDPOINT_ADDRESS_PROPERTY, stsURL);
requestContext.put(WSSecurityContext.TRUST_MANAGER,
new TrustManager() {
public boolean certificateCallback(X509Certificate[] chain, int validateErr) {
// need to validate if the server cert can be trusted
return true;
}
});
credList.add(new SAMLTrustCredentialProvider());
String username = "Alice";
String password = "weblogic1";
credList.add(new ClientUNTCredentialProvider(username.getBytes(), password.getBytes()));
// ClientBSTCredentialProvider
String defaultClientcert = "C:/projecten/workspace/11g_prod/saml1.1_ws/wsttest1/certs/Alice.cer";
String clientcert = System.getProperty("target.clientcert", defaultClientcert);
String defaultClientkey = "C:/projecten/workspace/11g_prod/saml1.1_ws/wsttest1/certs/Alice.prv";
String clientkey = System.getProperty("target.clientkey", defaultClientkey);
String defaultServerCert = "C:/projecten/workspace/11g_prod/saml1.1_ws/wsttest1/certs/Bob.cer";
String serverCert = System.getProperty("target.serverCert", defaultServerCert);
credList.add(new ClientBSTCredentialProvider(clientcert, clientkey, serverCert));
requestContext.put(WSSecurityContext.CREDENTIAL_PROVIDER_LIST, credList);
// Add your code to call the desired methods.
System.out.println(echoService.echo("Hello"));
} catch (Exception ex) {
ex.printStackTrace();
}
}
}
It is better to use OnBehalfOf mechanism for this type of use case.
ReplyDeleteOtherwise it is impossible for the STS to distinguish between the credentials from the end user and the ones from the proxy.
Modified the article to use WebLogic WebService Security Service relying party/asserting party:
ReplyDeletehttp://htotapally.blogspot.com/2010/08/web-service-security-wss-sender-vouches_06.html
Hi Edwin,
ReplyDeleteI have an ejb web service deployed on weblogic server 10.3.I want to use SAML Token to authenticate the web service.
I don't want to use the STS.A soap Ui is calling the webservice:
The sample request is:
129.124.101.57
flt262766
urn:oasis:names:tc:SAML:2.0:ac:classes:Password
How I can I achieve this./Can I use default key stores fo rtesting.It will be great help if u can give me straight steps to achieve the same.I am very new to these things
Hi,
ReplyDeleteDont know if you can do this with SOAPUI
you have more success with a java proxy client.
or this can help http://htotapally.blogspot.com/2010/08/web-service-security-wss-sender-vouches_06.html
thanks
Thanks for these detailed steps. I have a query. I have done all the steps mentioned mentioned in the document, however I am facing a problem in writing the "EchoServicePortClient" client. At line number 113, a string variable is declared to hold the client private key file path as shown below.
ReplyDelete[
...
String defaultClientkey = "C:/projecten/workspace/11g_prod/saml1.1_ws/wsttest1/certs/Alice.prv";
...
]
Could you please let me know, how to generate this .prv file?
Hi,
ReplyDeleteyou can download openssl or use the keytool.
or follow these steps http://biemond.blogspot.com/2009/06/ws-security-in-osb.html
thanks
Hello Edwin,
ReplyDeleteHow can we create the "EchoServicePortClient" client for a JAX-RPC web service client?
To be more specific, how can we get/set requestContext and other properties for JAX-RPC based web service client.
referecne:
http://biemond.blogspot.com/2009/10/securing-web-services-with-saml-sender.html
Thanks
Hi,
ReplyDeleteDont know exactly, I use jax-ws with OWSM but I think you can use the weblogic ws security profiles with jax-rpc.
Can't you use jax-ws
thanks
Hello Edwin,
ReplyDeleteWhat is the significance of "stsUntPolicy" defined in the client and can we avoid it as I have read someewhere client side policies are required only when policies are not exposed through WSDL. can we read policy from WSDL instead of passing it from client?
Thanks
[
ReplyDeleteHow can we create the "EchoServicePortClient" client for a JAX-RPC web service client?
To be more specific, how can we get/set requestContext and other properties for JAX-RPC based web service client.
...
]
found the Solution: use ANT with "generatePolicyMethods" set to true.
If you specify True, four flavors of a method called getXXXSoapPort() are added as extensions to the Service interface in the generated client stubs, where XXX refers to the name of the Web Service. Client applications can use these methods to load and apply local WS-Policy files, rather than apply any WS-Policy files deployed with the Web Service itself. Client applications can specify whether the local WS-Policy file applies to inbound, outbound, or both SOAP messages and whether to load the local WS-Policy from an InputStream or a URI.
Valid values for this attribute are True or False. The default value is False, which means the additional methods are not generated.
remaining properties of client can be set using stub itself.
[
ReplyDeleteWhat is the significance of "stsUntPolicy" defined in the client and can we avoid it as I have read someewhere client side policies are required only when policies are not exposed through WSDL. can we read policy from WSDL instead of passing it from client?
]
Answer:
If the security policy file of the Web Service specifies that the SOAP request must be encrypted, then the Web Services client runtime automatically gets the server’s certificate from the policy file that is attached to the WSDL of the service, and uses it for the encryption. If, however, the policy file is not attached to the WSDL, or the entire WSDL itself is not available, then the client application must use a client-side copy of the policy file. For more information refer http://download.oracle.com/docs/cd/E12840_01/wls/docs103/webserv_sec/message.html
Hi,
ReplyDeletefor the client it should be possible to read / import the WSDL. Open the WSDL and compare it with the client code.
thanks
Hi,
ReplyDeleteI'm trying to do a SOA invocation from SOAPUI. The composite has policy wss10_saml_token_with_message_protection_service_policy attached to it. Created, exported and imported keys as follows using JRE 1.7 (SKI):
Client:
C:\"Program Files"\Java\jre1.7\jre1.7.0\bin\keytool.exe -genkeypair -alias client_alias -keyalg "RSA" -sigalg "SHA1withRSA" -dname "cn=a, ou=b, o=c,c=US" -keypass password -keystore client.jks -storepass password -validity 5000
C:\"Program Files"\Java\jre1.7\jre1.7.0\bin\keytool.exe -exportcert -alias client_alias -file public_key.cer -keystore client.jks
Server: (Following were done in client machine itself and finally default-keystore.jks ftp-ed to server)
C:\"Program Files"\Java\jre1.7\jre1.7.0\bin\keytool.exe -genkeypair -alias server_alias -keyalg "RSA" -sigalg "SHA1withRSA" -dname "cn=a, ou=b, o=c,c=US" -keypass password -keystore default-keystore.jks -storepass password -validity 5000
C:\"Program Files"\Java\jre1.7\jre1.7.0\bin\keytool.exe -importcert -keystore default-keystore.jks -trustcacerts -alias server_alias1 -file public_key.cer
Invocation fails with following error stack:
at weblogic.servlet.internal.ServletRequestImpl.run(ServletRequestImpl.java:1454)
at weblogic.work.ExecuteThread.execute(ExecuteThread.java:207)
at weblogic.work.ExecuteThread.run(ExecuteThread.java:176)
Caused by: oracle.wsm.common.sdk.WSMException: InvalidSecurity : error in processing the WS-Security security header
at oracle.wsm.security.policy.scenario.executor.Wss10SamlWithCertsScenarioExecutor.receiveRequest(Wss10SamlWithCertsScenarioExecutor.java:197)
...........
at oracle.fabric.common.BindingSecurityInterceptor.processRequest(BindingSecurityInterceptor.java:94)
... 34 more
Caused by: oracle.wsm.security.SecurityException: WSM-00169 : Error decrypting the request message.
at oracle.wsm.security.policy.scenario.processor.Wss10MessageSecurityProcessor.decrypt(Wss10MessageSecurityProcessor.java:206)
...........
at oracle.wsm.security.policy.scenario.executor.Wss10SamlWithCertsScenarioExecutor.receiveRequest(Wss10SamlWithCertsScenarioExecutor.java:168)
... 43 more
Caused by: FAULT CODE: FailedCheck FAULT MESSAGE: The signature or decryption was invalid
at oracle.security.xmlsec.wss.WSSecurity.decrypt(WSSecurity.java:2382)
............
at oracle.dms.servlet.DMSServletFilter.doFilter(DMSServletFilter.java:136)
at weblogic.servlet.internal.FilterChainImpl.doFilter(FilterChainImpl.java:57)
... 9 more
Caused by: oracle.security.xmlsec.enc.XECipherException: Data must start with zero
at oracle.security.xmlsec.enc.XEEncryptedKey.decrypt(XEEncryptedKey.java:676)
at oracle.security.xmlsec.enc.XEEncryptedKey.getKey(XEEncryptedKey.java:788)
at oracle.security.xmlsec.wss.WSSecurity.decrypt(WSSecurity.java:2379)
... 46 more
Caused by: javax.crypto.BadPaddingException: Data must start with zero
at sun.security.rsa.RSAPadding.unpadOAEP(RSAPadding.java:393)
Not sure what is going wrong here.
Any help will be appreciated.
Thanks,
Vikas
Hello Edwin,
ReplyDeleteMy client application is a web application instead of standalone client. I don't want to hardcode the client certificate (required for signing) and server certificate (required for encryption) in my code. i.e. I don't want "new ClientBSTCredentialProvider(clientcert, clientkey, serverCert));". I want weblogic server to inject logged-in user certificate and private key and server certificate in the outgoing webservice call if it requires these message level securities. is it possible to configure this in weblogic?
Any help will be honestly appreciated.
Thanks, Sanjeev
Hi,
ReplyDeleteyep this is possible to do this from your weblogic container and use the keystores defined in this container. see my blog http://biemond.blogspot.com/2009/09/wsm-in-fusion-middleware-11g.html
I use for this the ADF Web Service Datacontrol where I can define the keystore in the Enterprise manager console.
And this also works from soa suite and OSB.
but I dont know how you can do this in a ws proxy client deployed on a wls container.
thanks
Hi,
ReplyDeleteplease can you first try it from jdeveloper so you know the keys are right and then move to soapui.
It can be that soapui or weblogic does something not right.
and add the debug parameters to weblogic to see all the output.
thanks
Hi,
ReplyDeleteI am creating saml token by using weblogic and I am following this blog I want you know that am i following the appropriate blog ?
Please reply.
if anyone have any idea about how to create saml using weblogic
plz let me know.
Hi,
ReplyDeleteit depends, are you talking about web applications or web services.
just click on the saml tag and you will see all the blogs.
thanks
Hi Edwin
ReplyDeleteI am talking about web services.
I am creating saml token through weblogic. I am following this blog (http://biemond.blogspot.com/2009/10/securing-web-services-with-saml-sender.html) . Am I following the appropriate blog? I am quite new to all these things .so please tell me step by step procedure for genrating saml Please Reply .
Hi,
ReplyDeleteWhat is your use case , do you need to protect your ws with a saml policy , and arrange the trust .
Or do you need to create a ws client which talks against a saml ws.
Do you need to use java or can you use OSB , SOA suite with OWSM .
Thanks
Hi Edwin,
ReplyDeleteI am documenting the requirement.
what we have to do is
we have to create some web service through weblogic that will take user credential(username pwd) and then it will genrate saml token.This saml token will go to the requested services. The saml token contains the URL(of requested servies) along with the credentials.It will act as a authenticater for the requested services. on the other side this saml token will be verified and if it is authentic. the access will be granted(we will be able to access services).
Thanx
Hi,
ReplyDeleteThen is this the right blog.
you need to create this sts service, the users authenticate with username password against this weblogic sts web service and get a signed saml token back.
There is a trust between this sts ws and the ws so it will except the ws request.
you can also use OpenSSO STS, this is not wls.
good luck
Hi Edwin,
ReplyDeleteThanks for this blog.
I see that the service is invoked (verified by logging).
But I get the following fault on the client side.
javax.xml.ws.soap.SOAPFaultException: Unable to add security token for identity
at com.sun.xml.ws.fault.SOAP11Fault.getProtocolException(SOAP11Fault.java:188)
at com.sun.xml.ws.fault.SOAPFaultBuilder.createException(SOAPFaultBuilder.java:108)
at com.sun.xml.ws.client.sei.SyncMethodHandler.invoke(SyncMethodHandler.java:119)
at com.sun.xml.ws.client.sei.SyncMethodHandler.invoke(SyncMethodHandler.java:89)
at com.sun.xml.ws.client.sei.SEIStub.invoke(SEIStub.java:118)
I have enabled SAMl and other security debugging, and could see following in WL logs
#### <[ACTIVE] ExecuteThread: '19' for queue: 'weblogic.kernel.Default (self-tuning)'> <> <> <> <1322120242683> ")
, initiator=Subject: 0
, resource=null, credType=SAML.Assertion.DOM>
#### <[ACTIVE] ExecuteThread: '19' for queue: 'weblogic.kernel.Default (self-tuning)'> <> <> <> <1322120242683>
Any idea what could be the issue?
Thanks,
Jackson
Hi,
ReplyDeletewhere did you get this error at what stage.
and what does it say at the client side.
thanks
Hi Edwin,
ReplyDeleteThanks for your response.
I get a SOAP fault at the client side
javax.xml.ws.soap.SOAPFaultException: Unable to add security token for identity
At the server side, I see following error message
not supported credential type SAML.Assertion.DOM
I could see that the service getting invoked and getting completed. Later, I was able to get the example working by applying the policy only in the request message.
What could I have done wrong?
Any idea how can I modify this example to use Holder-Of-Key profile?
Thanks,
Jackson
Hi,
ReplyDeleteI am getting the following error on the sts server side -
weblogic.wsee.security.wst.faults.RequestFailedException: Trust is unable to handle token type: http://docs.oasis-open.org/wss/oasis-wss-saml-token-profile-1.0#SAMLAssertionID
Any idea what the issue could be?
Hi,
ReplyDeleteStrange and not much output. Does the sts server validates the username.
what is your wls version. in my example I use a simple wls domain for the sts.
thanks
Edwin:
ReplyDeleteI adapted your post for SAML2.0 webservices security. I have an STS (on WL 10.3.5) service that authenticates a user using UNT, and then issues a SAML2.0 assertion for a web service that is deployed in another domain. What I see in the STS domain logs is that the SAML2.0 assertion gets generated successfully but the credential mapper does not seem to be forwarding the request on to the Web Service endpoint (along with the generated assertion) over to the second domain. I see no activity in the second WL domain's logs.
At the java client end I do get an "Unable to add token to identity" error. I have turned on debugging, but see no exceptions on either side (idp vs sp) that indicates a problem. I have configured the PKI, Credential Mapper, Service Provider Partner (within the STS/IDP domain) along with a PKI, SAML Auth, Identity Assertion Provider, Identity Provider Partner (within the SP domain).
Any suggestions are appreciated.
Hi,
DeleteI think the problem is in the client and there is a mismatch in the keys or policies at the client ( i think in the initial request there is only username/ password and sts responds with keys ). Maybe a jdeveloper http analyzer in between can help.
good luck
Edwin thank you for the reply.
DeleteI am not using the same certs that you recommended in your worked out example. I have the following setup as far as certs are concerned.
STS/IDP (first WL domain, first box)
* IDP identity cert
* IDP trust cert - that has the client public key imported
* PKI - uses the client cert
Web Service/SP (second WL domain, second box)
* SP identity cert
* SP trust cert - that has the client public key imported
* PKI - uses the client cert
* SamlAuth - SAML Authentication Provider
* WS Identity Provider Partner - created within the SAML Identity Assertion Provider in SAML2.0 - this imports the STS/IDP's public cert (as part of the Assertion Signing Cert).
Standalone Client (Your code)
* Client identity cert
* Client trust - imports the STS/IDP's public key
* Other than that my client code is pretty much the same as yours
A few questions:
a. I assume this is an SP initiated SAML use case - true?
b. When you call the SP WS, you provide all the information of the STS via the BindingProvider, I assume that, that is what gets the STS involved in producing the SAML assertion.
Java Client -> SP WS -> STS WS (produce token) -> SP WS (execute method) - is this accurate?
c. However, the response posted by the STS does not seem to even come back to the SP WS - not sure why.
Edwin:
DeleteTo answer your earlier question - yes on initial request, there is only a username/password policy (targeted at the STS WS and that user is available within the STS WL domain). I see the authentication succeeding and SAML assertion creation. It almost appears that the STS is posting the response back to something other than the SP WS.
Thank you.
Hi,
DeleteIt has been a while but the sts should post back to the client and use the cert of the sts and the username of the client in the sp ws. Try to add a lot of traffic monitoring , use the same certs as mine and make first my example working
Good luck
Hi Edwin
ReplyDeleteExcellent blog. I am trying to simulate the similar stuff using OSB for the Oracle Human workflow tasks. I am looking for a sample to run the task services using SAML binding port.
1) Created a basic OSB proxy pointing to TaskQueryService SAML binding port for a queryTasks operation.
2) Attached "oracle/wss10_saml20_token_client_policy" on the proxy.
3) For testing the proxy, I believe I need an SAML assertion to be present up in SOAP header. So, I have created another testProxy which calls TaskQueryService direct binding (non-saml based) and attached same SAML policy, same as the other one and ran the test to generate a SAML assertion. It works fine and I get the response back with SAML assertion in the request message SOAP header.
I have picked up this saml assertion generated from the SOAP header and copied it in the SOAP header of test client for SAML based test proxy.
But it throws the below error:
"invalidsecurity error in processing the ws-security security header"
I was not successful in generating the SAML assertion from SOAP-UI to test the similar scenario so I followed the route of OSB test client.
Can you please provide me the sample xml message for testing the tasking services using SAML. Also, please let me know the correct steps for testing SAML stuff from OSB.
Many Thanks
Hi Edwin
DeleteAny suggestions on the authentication based on the SAML binding for Oracle Workflow task services and the answers to my above query?
Thanks
Khad
Hi,
Deletedid you follow this http://biemond.blogspot.nl/2011/08/do-saml-with-owsm.html this blog explains it all
make an osb proxy with username token policy which call a BS with saml client policy ( the username is used for the saml token ) and then call the Human WF services
thanks
Hi Edwin
DeleteMany thanks for your suggestions. I have tried your suggested approach and it works from OSB proxy(user-name-token) to BS(saml-client-token). But going forward, the client in my case is going to be ADFS .Net application which will be calling an OSB proxy on a service layer. Before the ADFS client-OSB authentication process, I would like to test this OSB-SOA authentication and it was the reason why I have raised a query above. But
I am still not clear on the how to pass the client credentials as encrypted to the BS as rather than clear text for the Human workflow task services.
For this, I have posted a query on Oracle support forums but no replies so far. Please kindly have a look.
https://forums.oracle.com/forums/thread.jspa?threadID=2490514&tstart=0
Probably, I am making a mistake in my approach.
Can you please suggest me the correct approach.
Cheers
Khad
Hi,
DeleteI think saml owsm policy only works on the OSB proxy and not passed on ( no identity propagation) you should use a new owsm policy like signature and message protection between osb and soa suite.
Thanks
Hi,
ReplyDeleteI have a confounding error message being returned from the STS bootstrap (I know I have not accessed the bootstrap ws using ssl, could this be causing the problem?):
java.io.IOException: Received a response from url: http://172.16.35.225:7010/SAMLOsts12/StsUntService with HTTP status 200 and SOAP content-type: null.
I used wireshark to check the messages and true enough, the message received from the weblogic is empty. On the other hand, the body of the request message has only security information and doesn't actually pass anything to the dummy function.
Thanks in advance.
Tried with SSL. Same result.
DeleteHi,
DeleteI don't have any ideas what your problem can be. You can set the logging to trace and maybe this gives you some more information, what really happens.
Thanks
Hi Edwin,
ReplyDeleteWe are currently integrating with Oracle Serity Token Service by calling issueToken operation
from this wsdl(http://hostname:14100/sts/wss11user?WSDL) to get the token from STS. STS does return the
SAML token for us. On the same WSDL there is a validate token operation that we cant see to get it to work.
Actually we dont know the structure of the payload we need to send to STS for a SAML token validation.
Any help will be appreciated.
Regards
Emmanuel
Edwin,
ReplyDeleteFirst, thanks for this post (so long ago!)...
I hope you are still willing to answer questions about it.
In your text, there are two services you have to enter in as config parameters, but, you don't say or show what they are. These are displayed in the images 10 of 20 and 16 of 20. Can you please clarify what these services are, or, how I obtain the url to enter?
Also, another user mentioned the ".prv" file you refer to in the client code. You said the answer could be found in another blog post. I read that post and was still curious which of the files is actually being referred to - would it be client.key, client.pem, or, client.p12.
Lastly, I notice this service uses the saml1.1 policy. Is all of this still applicable to the saml2.0 policy? I am required to use saml2...
I would greatly appreciate your help. I have been trying to get a saml secured webservice working for some time before I (finally) ran across this post.
Thanks!
Stan
Hi Edwin,
ReplyDeleteI am not getting how to generate .prv file
I went through the link (http://biemond.blogspot.com/2009/06/ws-security-in-osb.html) but could not get the step to generate .prv file..
Could you please advise on how to generate .prv file...
Also, I have the certificate and key generate using below command:
java utils.CertGen -certfile ServerCACert -keyfile ServerCAKey -keyfilepass password -selfsigned -e somebody@xxxx.com.au -ou FOR-DEVELOPMENT-ONLY -o XXXX -l MELBOURNE -s VIC -c AU -cn 127.0.0.1
But the key is in .der and .pem format...
And when I use this key in proxy client code it gives me below error:
java.security.spec.InvalidKeySpecException: java.security.InvalidKeyException: invalid key format
at sun.security.rsa.RSAKeyFactory.engineGeneratePrivate(RSAKeyFactory.java:200)
at java.security.KeyFactory.generatePrivate(KeyFactory.java:342)
at weblogic.wsee.security.util.CertUtils.getPKCS8PrivateKey(CertUtils.java:226)
at weblogic.wsee.security.util.CertUtils.getPKCS8PrivateKey(CertUtils.java:207)
at weblogic.wsee.security.bst.ClientBSTCredentialProvider.(ClientBSTCredentialProvider.java:141)
at ws.EchoServiceSAML2PortClient.main(EchoServiceSAML2PortClient.java:127)
Please help me out...
Regards,
Sanket